Advanced Usage
Custom Actions
You can define actions through code.
For more flexible and diverse test writing, you can define actions using Playwright-based code.
1. Creating Custom Actions
You can add a custom action by clicking the [+] button > [Add Custom Action] button at the top right of the [Test Steps] panel in the Reliv Editor.
Click the [>] (detail view) button on the custom action to write the code.
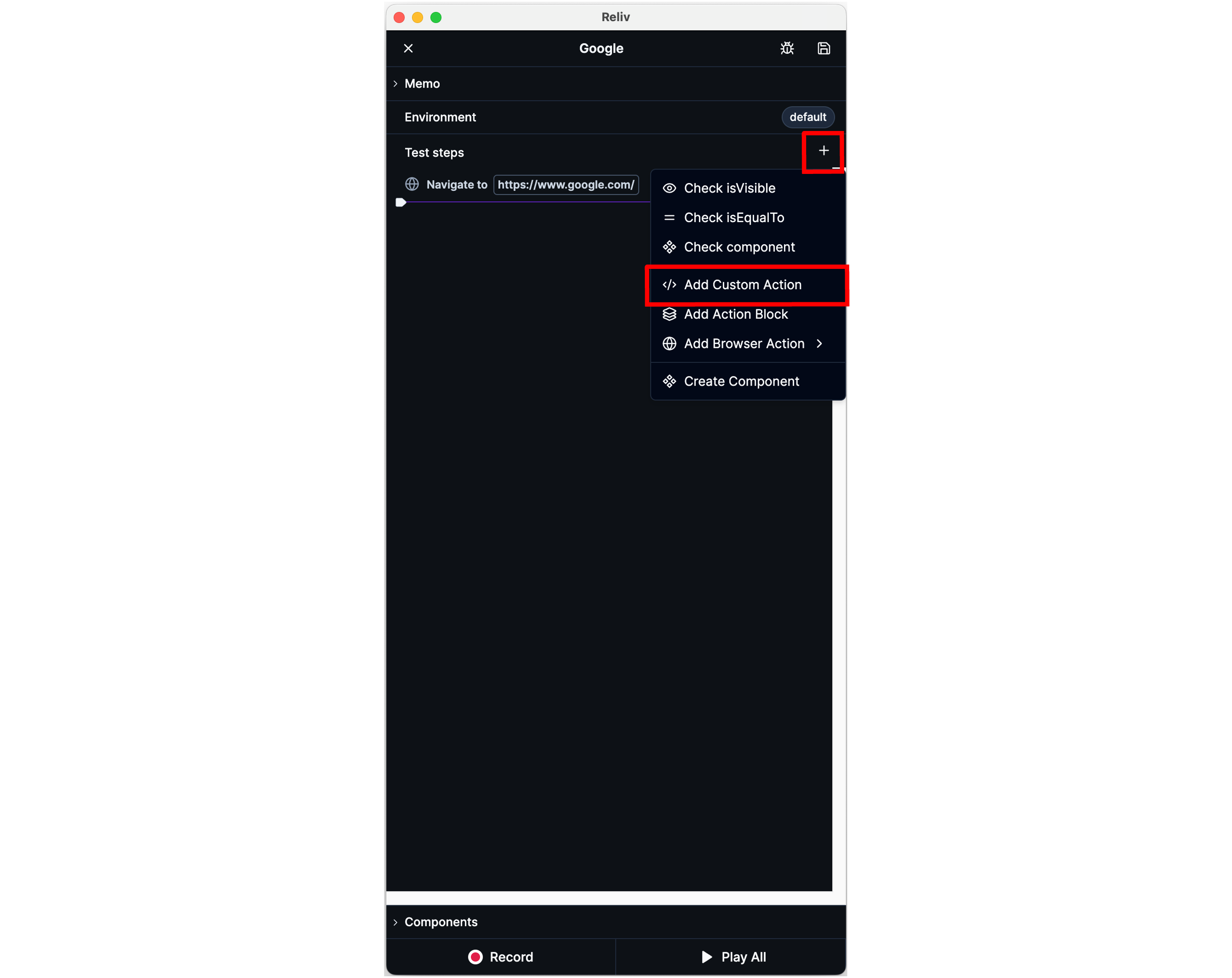
2. Writing Custom Action Code
The following Playwright objects can be used within custom code:
For detailed API descriptions, please refer to the Playwright Docs.
3. Custom Action Code Examples Reference
Click
await page.getByRole("button", { name: "Buy" }).click();
await page.locator("nav").getByRole("link", { name: "Tutor" }).click();
Fill
await page.locator(`input[value="Reliv one"]`).fill("Reliv two");
Hover
await page
.locator("#AdminListItem")
.filter({ hasText: "Reliv Test Channel" })
.hover();
Navigate
await page.goto("https://google.com/");
Check URL
await expect(page).toHaveURL(/https:\/\/google\.com\/products/);
Check Clipboard
const clipboardText = await page.evaluate("navigator.clipboard.readText()");
// Check if the clipboard value contains the target text
expect(clipboardText).toContain("text");
// Check if the clipboard value matches exactly
expect(clipboardText).toEqual("equalText");
Handling iframes
await page
.locator(`iframe[title="Modal Message"]`)
.frameLocator(":scope")
.locator(`button.bz-close-btn`)
.click();
Handling popup page
const pagePromise = context.waitForEvent("page");
const googleBtn = page.getByAltText("Google Login").click();
const newPage = await pagePromise;
await expect(newPage).toHaveTitle(/Google/);
await newPage.getByLabel(/Email/).fill("reliv@gmail.com");
await newPage.getByRole("button", { name: "Next" }).click();
await newPage.getByLabel("Enter your password").fill("testtest");
await newPage.locator("#passwordNext").click();
Using for loop
const trialLinks = await page.locator("#Card");
expect(trialLinks).toBeVisible();
const linkCount = await trialLinks.count();
for (let i = 0; i < linkCount; i++) {
await trialLinks.nth(i).click();
await expect(page).toHaveURL(/https:\/\/google\.com\/products/);
await page.goBack();
}
API Login
await page.evaluate(async () => {
// Set your API login URL, username, and password
const loginUrl = "https://your-api-domain.com/api/login";
const payload = {
username: "testuser",
password: "testpassword",
};
const response = await fetch(loginUrl, {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify(payload),
});
const data = response.json();
// Save token to localStorage (change this to your own logic)
if (data.token) {
localStorage.setItem("token", data.token);
}
});
// refresh (if needed)
await page.reload();